[Option 2]
Use a secondary timer that monitors a flag that the websocket.onmessage() handler sets. Run this at say 10ms.

DriverTimer's onEvent 'getDriverData' {}Slot is now as follows:
native()
{
flag = 0;
ws.binaryType = "arraybuffer";
ws.send("websocket_command");
ws.onmessage = function(evt) {
onMessage(evt);
};
function onMessage(evt) {
var data = evt.data;
var dv = new DataView(data);
ref_co2 = dv.getFloat32(0);
flag = 1;
}
}
Note we dont pass any locals into the native(). They have to be defined as javascript globals using an Inline block, otherwise they wont be available in both slots for each timer.
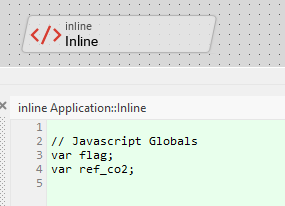
The secondary timer monitor, which looks for a flag that indicates these globals were stuffed, launches a WebsocketProcessor slot. This slot moves the javascript vars, now with data, into Chora locals to then be sent into an update {}method:
// locals
var int8 l_flag = 0;
var float l_ref_co2;
native(l_ref_co2)
{
// websocket.onmessage() event handler just filled our global vars, so stuff locals
l_flag = flag;
l_ref_co2 = ref_co2;
flag = 0;
}
// websocket.onmessage() event handler just filled our global vars, so pass locals on
if(l_flag == 1)
{
IRGA_update(l_ref_co2);
}