Hello Daniel,
To achieve the desired endless behavior you can modify the provided example. Following are the necessary steps:
Step 1: Navigate to the class Application::List. There you see two Horizontal List views: ListR and ListL. Select the views and change their properties Endless to the value true as shown in the screenshot below:
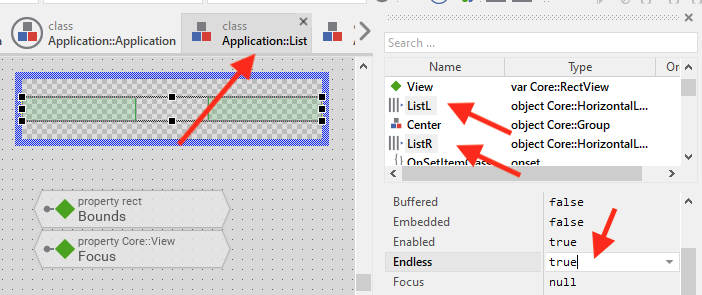
Step 2: In the class Application::List open the method UpdateViewState and replace its code with the following version. This enhances the original implementation by special handling of the overflow when the list scrolls endless:
super( aState );
if ( ItemClass == null )
return;
// Calculate the size of the center area and the of the list lying to the left of it.
var point bounds = Bounds.size;
var int32 widthCenter = CenteredItemSize.x + RegularItemSize.x * 2;
var int32 widthListL = ( bounds.x - widthCenter ) / 2;
// Calculate the scroll-offsets for the both lists.
ListL.ScrollOffset = ScrollOffset + widthListL + RegularItemSize.x;
ListR.ScrollOffset = ScrollOffset - RegularItemSize.x * 2;
// Calculate the difference to maximaly enlarge the item in the center compared to the
// size of a regular item. Calculate also the scroll offset expressed within the range
// of a single item. This means, 'offset' will lie in 0 .. (RegularItemSize.x - 1).
var point maxDelta = CenteredItemSize - RegularItemSize;
var int32 offset = 0;
if ( ScrollOffset >= 0 ) offset = RegularItemSize.x - ( ScrollOffset % RegularItemSize.x );
else offset = -ScrollOffset % RegularItemSize.x;
// Knowing the scroll offset within a single item, the resulting enlargement of the
// item in the center area.
var int32 deltaX = ( offset * maxDelta.x ) / RegularItemSize.x;
var int32 deltaY = ( offset * maxDelta.y ) / RegularItemSize.x;
array point size[4];
// Now calculate the size of the items in the center area. To simplify the application
// case we work with up to 4 items within the center area.
size[0] = RegularItemSize;
size[1] = point( RegularItemSize.x + maxDelta.x - deltaX,
RegularItemSize.y + maxDelta.y - deltaY );
size[2] = point( RegularItemSize.x + deltaX, RegularItemSize.y + deltaY );
size[3] = RegularItemSize;
// Now get the number of the item at the left edge of the center area.
var int32 firstItem = 0;
var int32 i;
if ( ScrollOffset >= 0 ) firstItem = -2 - ( ScrollOffset / RegularItemSize.x );
else firstItem = -1 - ( ScrollOffset / RegularItemSize.x );
// Process the items in the center area. Show/hide the items if there is no content
// assocuated to them. Move and resize the itmes.
for ( i = 0; i < centerItems.size; i = i + 1 )
{
// Derive the number of the item and determine the view used in the center area to
// represent the item.
var int32 item = firstItem + i;
var Core::RectView view = (Core::RectView)Center.GetViewAtIndex( i );
if ( item < 0 )
item = NoOfItems - ( -item % NoOfItems );
item = ( item + NoOfItems ) % NoOfItems;
// Is there a content for this item available?
if (( item >= 0 ) && ( item < NoOfItems ))
{
// The view is not valid. Reload the view.
if ( item != centerItems[i])
{
Item = item;
View = view;
signal OnLoadItem;
Item = -1;
View = null;
// Remember that the 'i' view has been loaded with the content of the item
// 'item'. In this manner avoid the views to reload its content permanently.
centerItems[i] = item;
}
// Ensure that the view is visible.
view.ChangeViewState( Core::ViewState[ Visible ], Core::ViewState[]);
}
// No associated content - hide the view.
else
view.ChangeViewState( Core::ViewState[], Core::ViewState[ Visible ]);
// The vertical position to arrange the item centered.
var int32 vertPos = ( bounds.y - size[i].y ) / 2;
// Arrange the item at the calculated offset position. Assign the item its new
// size.
view.Bounds = rect( -offset, vertPos, -offset + size[i].x, vertPos + size[i].y );
// The position for the next item. It should follow immediately.
offset = offset - size[i].x;
}
Step 3: In the class Application::List open the method onStartSlideSlot and replace its code with the following version. This changes the original implementation to provide an 'endless' (in fact 32k Pixel large) scroll area:
// Allow the user to scroll ~32 k pixel left/right
var int32 offset = 32000 - ( 32000 % pure RegularItemSize.x );
// Initialize the slide handler
SlideHandler.Offset = point( pure ScrollOffset, 0 );
SlideHandler.MinOffset = point( pure ScrollOffset - offset, 0 );
SlideHandler.MaxOffset = point( pure ScrollOffset + offset, 0 );
That is sufficient to enhance the fancy list with the endless functionality.
Nevertheless, the referenced example demonstrates just one possible application case. In practice, the behavior of such fancy list is always very individual to the expected UI design. With this I want to say, that with Embedded Wizard you get the framework with many components to assemble the GUI application. Individual desires expect however individual adaptations.
I hope it helps you further,
Bets regards
Paul Banach