I designed an animation to make the label of guage move evenly on the guage according to the ring path.
The final effect is to change from Figure 1 to Figure 2.
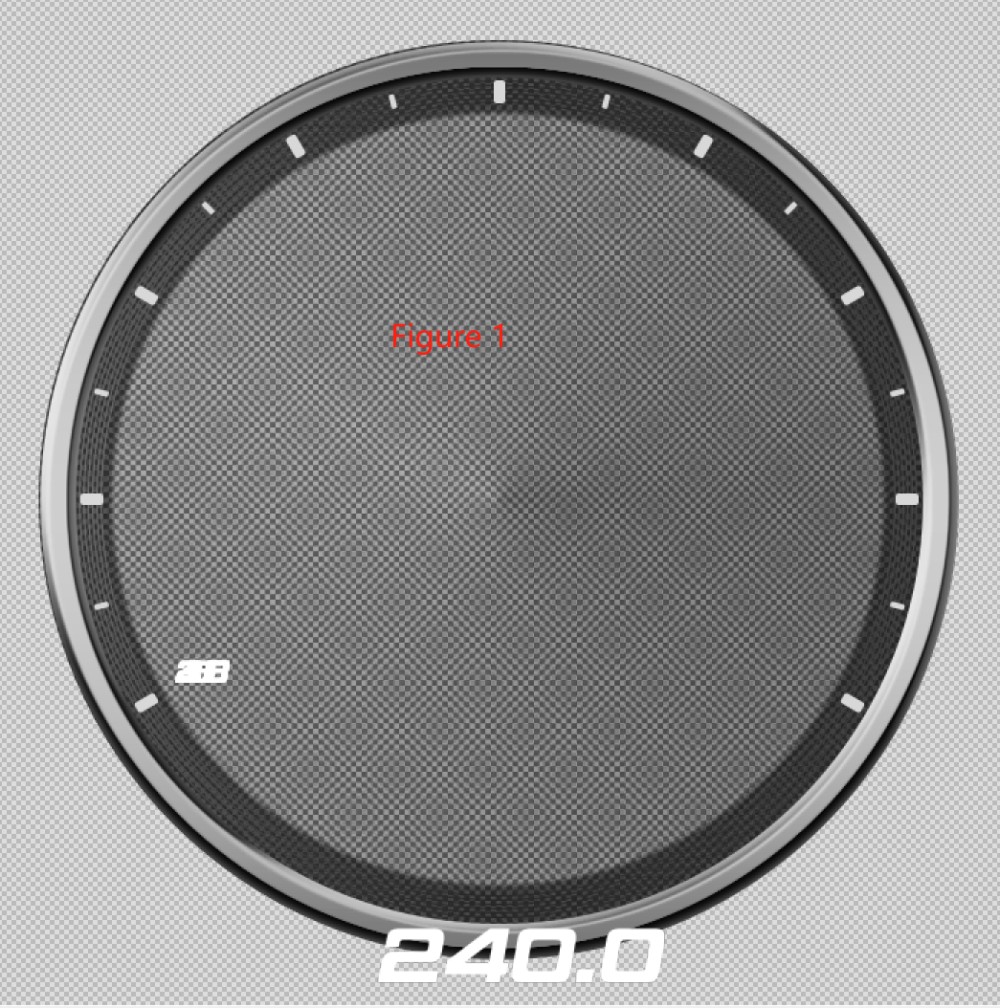
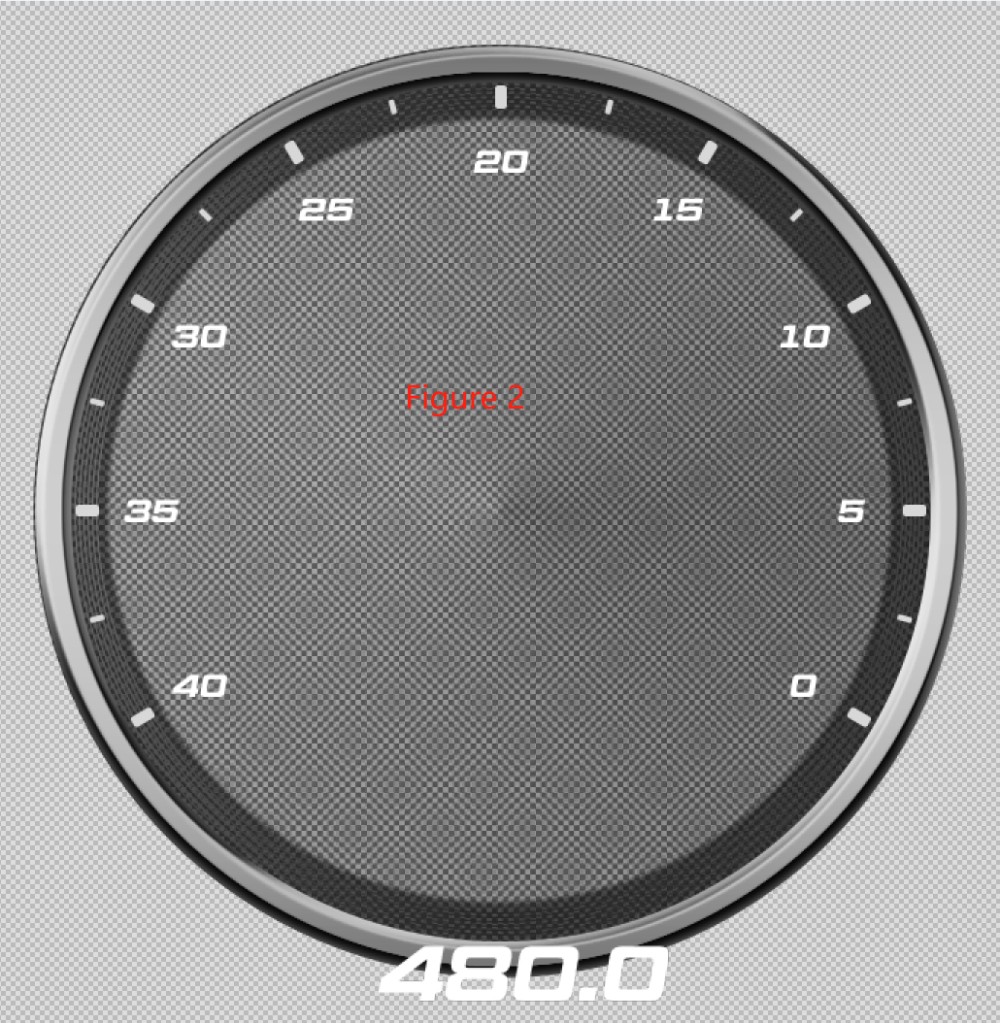
I made some changes based on the dashboard demo.
I rewrote the refresh test to get the effect I wanted.
The code for refresh is as follows:
// Calculate the angle between each text label
var float deltaAngle = ( endAngle - startAngle ) / float( labels.size - 1 );
var Effects::FloatEffect TempFloatEffect;
// Adjust and position each text label
var int32 i = 0;
while ( i < labels.size )
{
if ( Clockwise )
{
labels[ i ].String = string( i * increment );
aAngleArray[i] = startAngle + float(i) * deltaAngle;
TempFloatEffect = new Effects::FloatEffect;
if( i == 0)
{
TempFloatEffect.Value1 = aAngleArray[0];
TempFloatEffect.Value2 = aAngleArray[0];
}
else
{
TempFloatEffect.Value1 = aAngleArray[i-1];
TempFloatEffect.Value2 = aAngleArray[i];
}
TempFloatEffect.OnAnimate = Animation1;
TempFloatEffect.CycleDuration = 500;
postsignal TempFloatEffect.StartEffect;
}
else
{
labels[ i ].String = string( (labels.size - i - 1) * increment );
aAngleArray[i] = startAngle + float(i) * deltaAngle;
TempFloatEffect = new Effects::FloatEffect;
if( i == 0)
{
TempFloatEffect.Value1 = aAngleArray[0];
TempFloatEffect.Value2 = aAngleArray[0];
}
else
{
TempFloatEffect.Value1 = aAngleArray[0];
TempFloatEffect.Value2 = aAngleArray[i];
}
TempFloatEffect.OnAnimate = Animation1;
TempFloatEffect.NoOfCycles = 1;
TempFloatEffect.CycleDuration = 500;
postsignal TempFloatEffect.StartEffect;
}
i = i + 1;
}
The code for Animation1 is shown below:
sender; /* the method is called from the sender object */
var Effects::FloatEffect TempFloatEffect = (Effects::FloatEffect) sender;
var float deltaAngle = ( endAngle - startAngle ) / float( labels.size - 1 );
var int32 i = ( TempFloatEffect.Value2 - startAngle ) / deltaAngle;
var int32 dist = radius;
var int32 offset = 0;
var float aAngle = TempFloatEffect.Value;
if( i == labels.size - 1)
Text.String = string(aAngle);
// Calculate the label center
var point labelCenter = point( labels[ i ].Bounds.w / 2, labels[ i ].Bounds.h / 2 );
if ( aAngle > 360.0 )
aAngle = aAngle - 360.0;
// Draw values which can be divided by 20 with 10px less distance to the center
//if ( aNumber % 20 == 0 )
// aDistance = aDistance - 10;
// Add more distance for number with three or more digits
//if ( aNumber / 100 >= 1 )
// offset = 0;
//else
// offset = 10;
// Calculate the position of the label
labels[ i ].Bounds.origin = Bounds.orect.center - labelCenter
+ point( int32( float( radius + offset ) * math_cos( aAngle - 90.0 ) ), int32( float( radius + offset ) * math_sin( aAngle - 90.0 ) ) );
After my test, I found that the position of label was inconsistent each time (as shown in Figure 3 and Figure 4). The final reason is that when FloatEffect ends, FloatEffect.Value is not equal to FloatEffect.Value2 and is a random value.


So my question is does my animation idea work and what is the reason for this random effect?